DF: Benchmark Problems for CEC2018 Competition on Dynamic Multiobjective Optimisation¶
The problem suite is implemented based on [49].
DF1¶
[1]:
import numpy as np
from pymoo.problems.dynamic.df import DF1
from pymoo.visualization.scatter import Scatter
plot = Scatter()
for t in np.linspace(0, 10.0, 100):
problem = DF1(time=t)
plot.add(problem.pareto_front(), plot_type="line", color="black", alpha=0.7)
plot.show()
[1]:
<pymoo.visualization.scatter.Scatter at 0x107f30aa0>
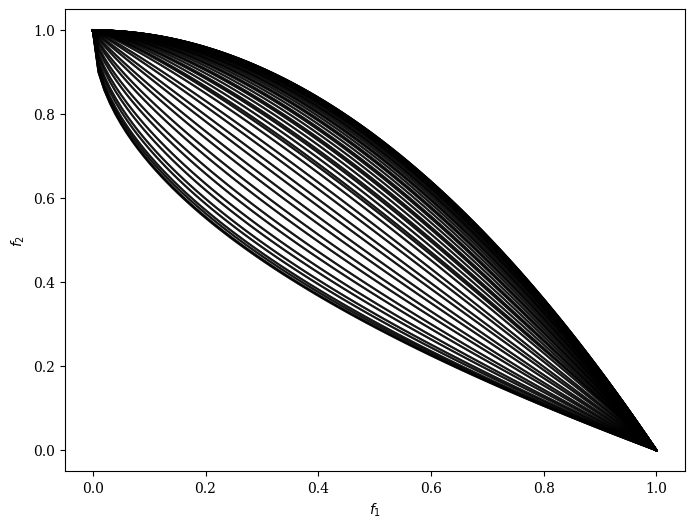
DF2¶
[2]:
from pymoo.problems.dynamic.df import DF2
plot = Scatter()
for t in np.linspace(0, 10.0, 100):
problem = DF2(time=t)
plot.add(problem.pareto_front(), plot_type="line", color="black", alpha=0.7)
plot.show()
[2]:
<pymoo.visualization.scatter.Scatter at 0x112285430>
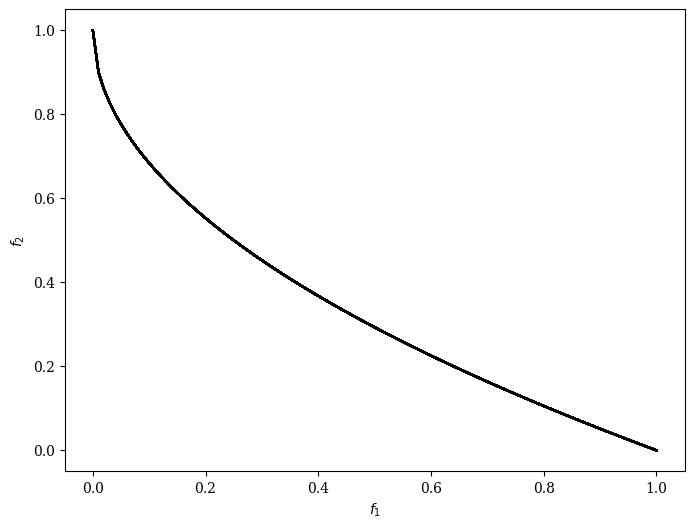
DF3¶
[3]:
from pymoo.problems.dynamic.df import DF3
plot = Scatter()
for t in np.linspace(0, 10.0, 100):
problem = DF3(time=t)
plot.add(problem.pareto_front(), plot_type="line", color="black", alpha=0.7)
plot.show()
[3]:
<pymoo.visualization.scatter.Scatter at 0x104244290>
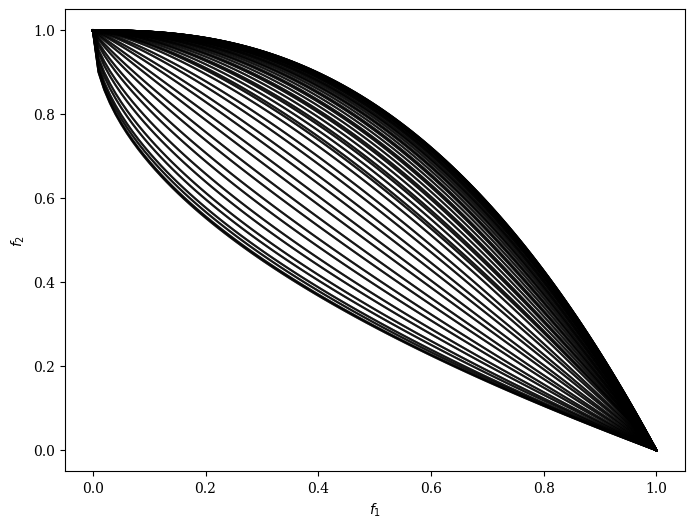
DF4¶
[4]:
from pymoo.problems.dynamic.df import DF4
plot = Scatter()
for t in np.linspace(0, 10.0, 100):
problem = DF4(time=t)
plot.add(problem.pareto_front() + 2*t, plot_type="line", color="black", alpha=0.7)
plot.show()
[4]:
<pymoo.visualization.scatter.Scatter at 0x1127197f0>
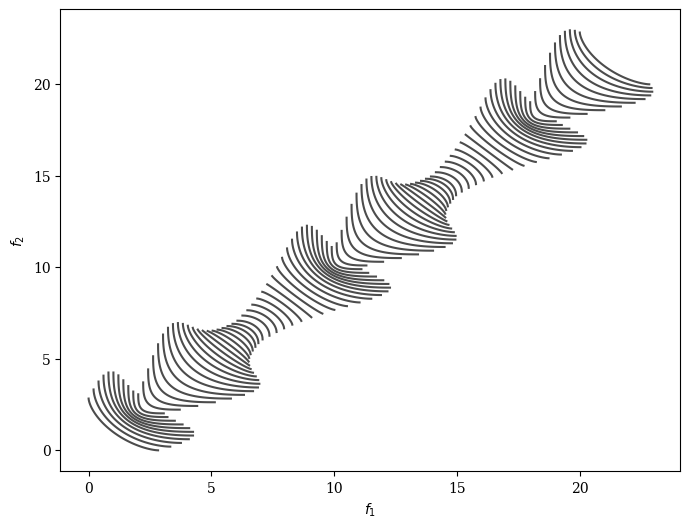
DF5¶
[5]:
from pymoo.problems.dynamic.df import DF5
plot = Scatter()
for t in np.linspace(0, 2.0, 100):
problem = DF5(time=t)
plot.add(problem.pareto_front(n_pareto_points=300) + 2*t, plot_type="line", color="black", alpha=0.7)
plot.show()
[5]:
<pymoo.visualization.scatter.Scatter at 0x11269e270>
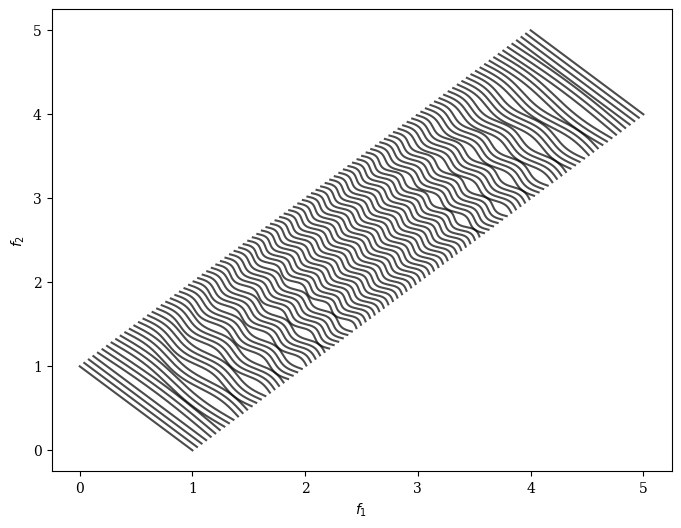
DF6¶
[6]:
from pymoo.problems.dynamic.df import DF6
plot = Scatter()
for t in np.linspace(0, 2.0, 100):
problem = DF6(time=t)
plot.add(problem.pareto_front(), plot_type="line", color="black", alpha=0.7)
plot.show()
[6]:
<pymoo.visualization.scatter.Scatter at 0x112946150>
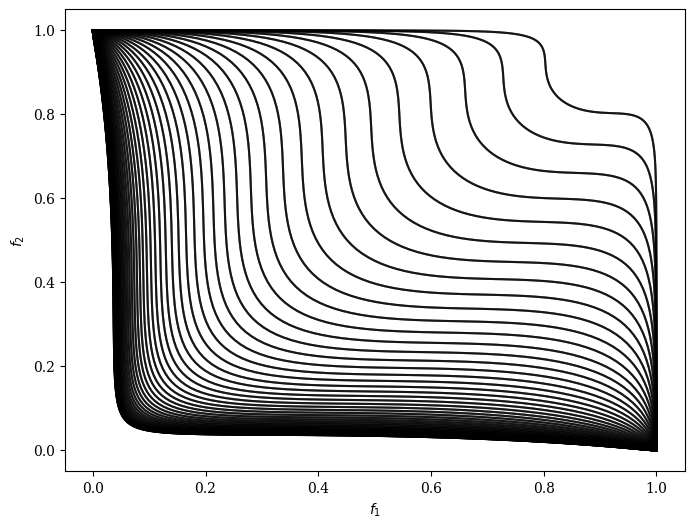
DF7¶
[7]:
from pymoo.problems.dynamic.df import DF7
plot = Scatter()
for t in np.linspace(0, 1.0, 20):
problem = DF7(time=t)
plot.add(problem.pareto_front() + 2*t, plot_type="line", color="black", alpha=0.7)
plot.show()
[7]:
<pymoo.visualization.scatter.Scatter at 0x1128cde80>
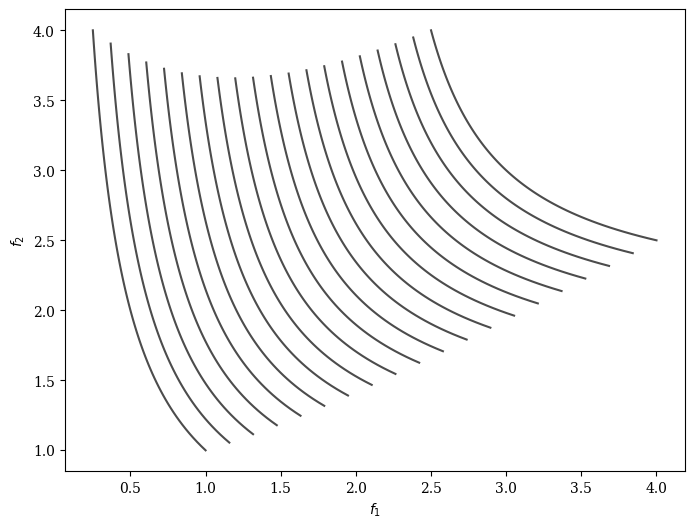
DF8¶
[8]:
from pymoo.problems.dynamic.df import DF8
plot = Scatter()
for t in np.linspace(0, 2.0, 20):
problem = DF8(time=t)
plot.add(problem.pareto_front() + 4*t, plot_type="line", color="black", alpha=0.7)
plot.show()
[8]:
<pymoo.visualization.scatter.Scatter at 0x11273fc50>
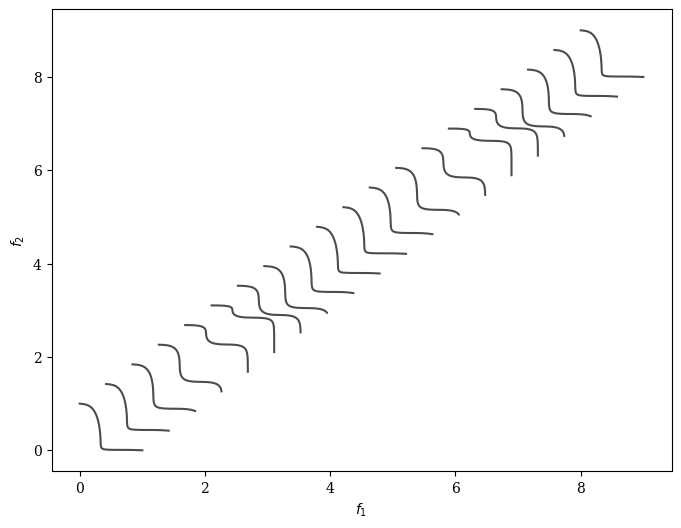
DF9¶
[9]:
from pymoo.problems.dynamic.df import DF9
plot = Scatter()
for t in np.linspace(0, 2.0, 20):
problem = DF9(time=t)
plot.add(problem.pareto_front() + 2*t, plot_type="line", color="black", alpha=0.7)
plot.show()
[9]:
<pymoo.visualization.scatter.Scatter at 0x1128cc3b0>
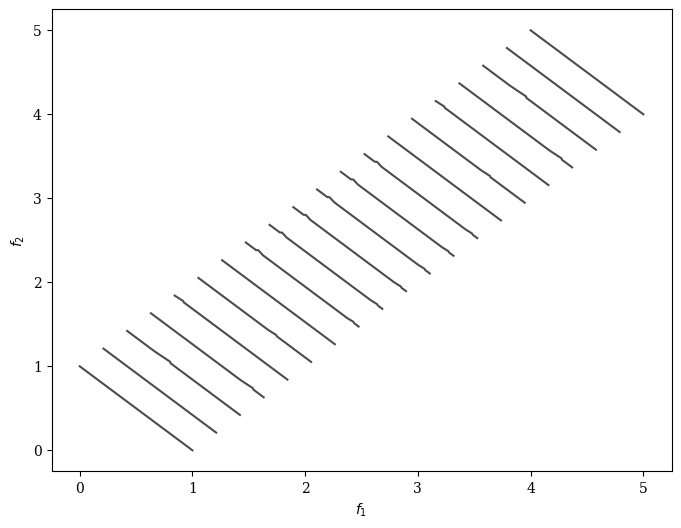
DF10¶
[10]:
from pymoo.problems.dynamic.df import DF10
import matplotlib.pyplot as plt
for t in [0.0, 1.0, 1.5, 2.0]:
plot = Scatter()
problem = DF10(time=t)
plot.add(problem.pareto_front() + 2*t, plot_type="line", color="black", alpha=0.7)
plot.do()
plt.show()
print("DONE")
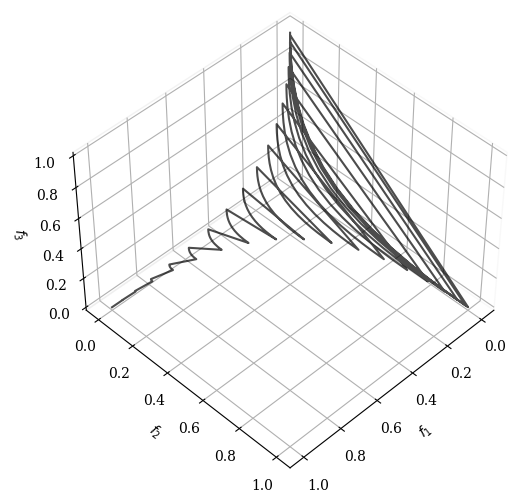
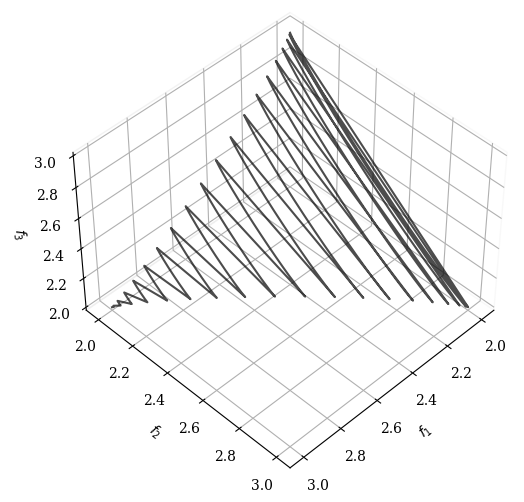
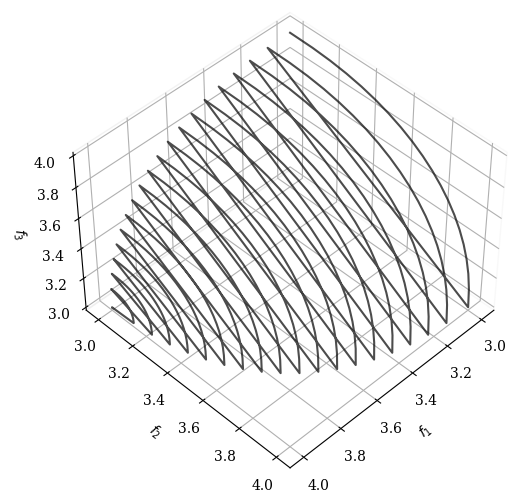
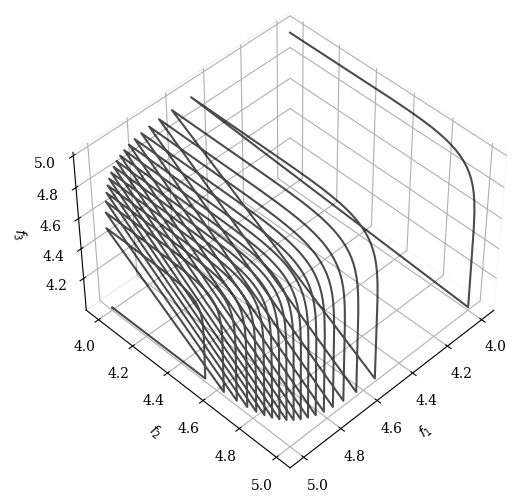
DONE
DF11¶
[11]:
from pymoo.problems.dynamic.df import DF11
import matplotlib.pyplot as plt
for t in [0.0, 1.0, 1.5, 2.0]:
plot = Scatter()
problem = DF11(time=t)
plot.add(problem.pareto_front() + 2*t, plot_type="line", color="black", alpha=0.7)
plot.do()
plt.show()
print("DONE")
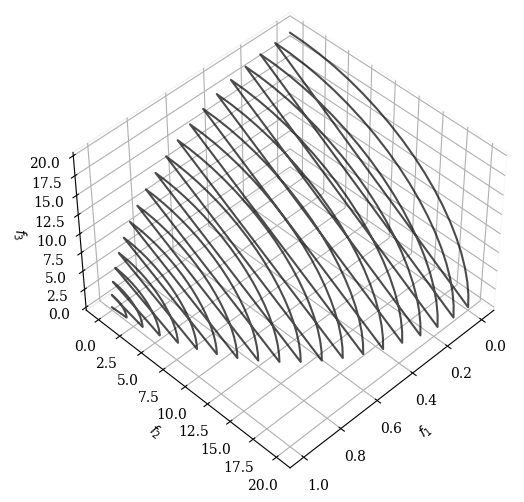
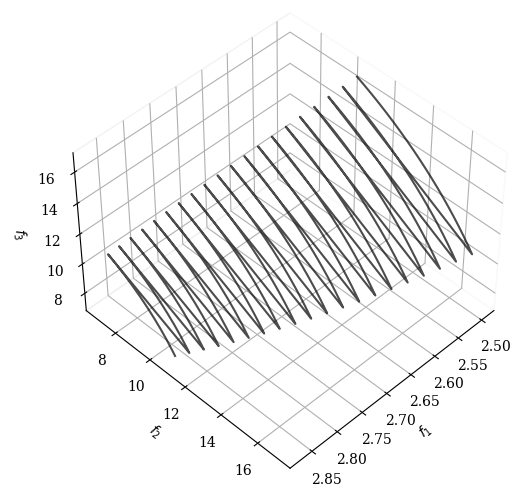
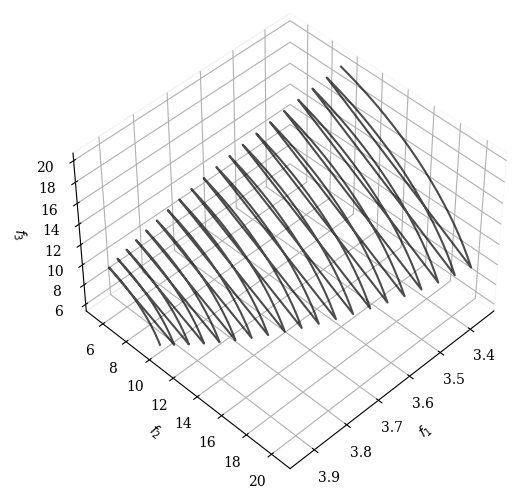
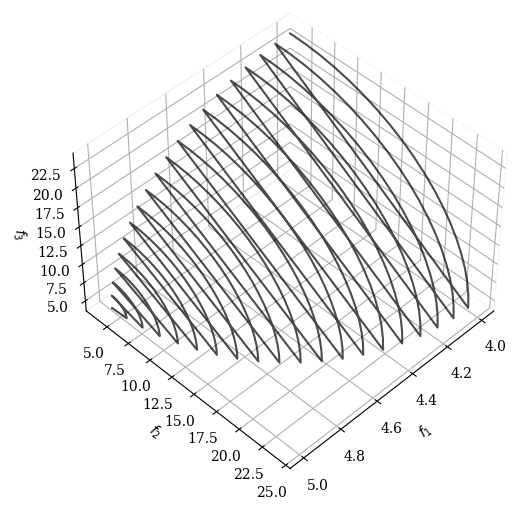
DONE
DF12¶
[12]:
from pymoo.problems.dynamic.df import DF12
import matplotlib.pyplot as plt
for t in [0.0, 0.1, 0.2]:
plot = Scatter()
problem = DF12(time=t)
plot.add(problem.pareto_front() + 2*t, color="black", alpha=0.7)
plot.do()
plt.show()
print("DONE")
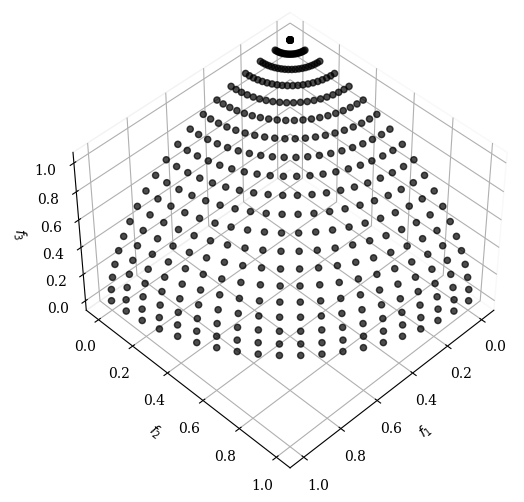
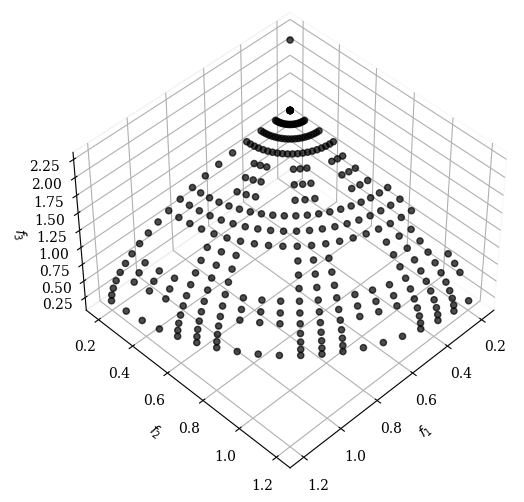
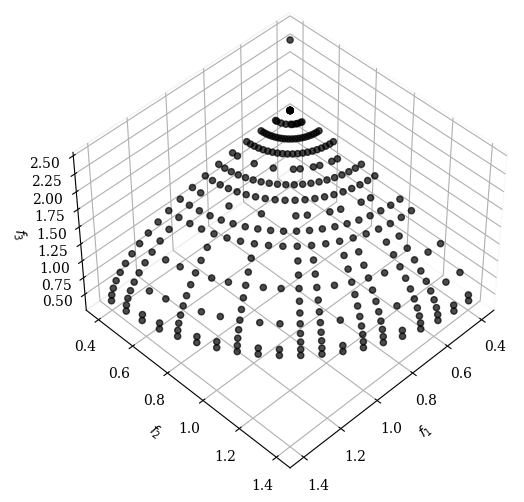
DONE
DF13¶
[13]:
from pymoo.problems.dynamic.df import DF13
import matplotlib.pyplot as plt
for t in [0.0, 0.2, 0.3, 0.4]:
plot = Scatter()
problem = DF13(time=t)
plot.add(problem.pareto_front() + 2*t, color="black", alpha=0.7)
plot.do()
plt.show()
print("DONE")
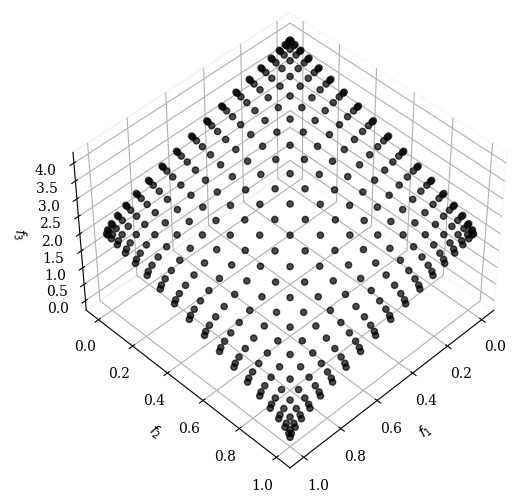
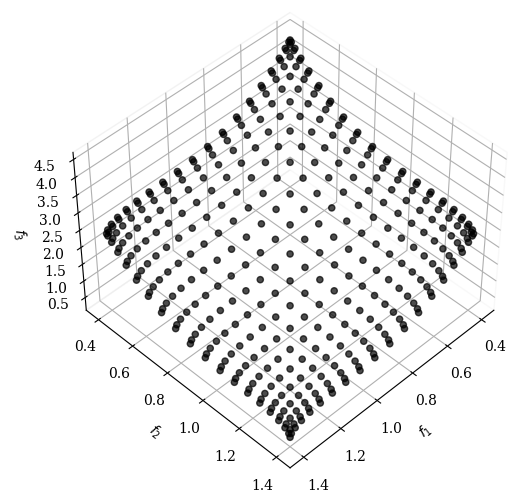
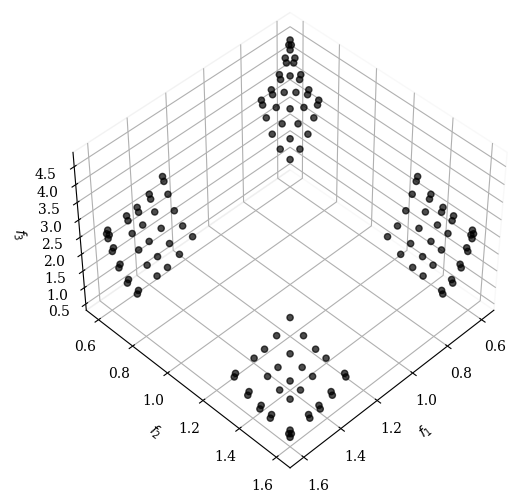
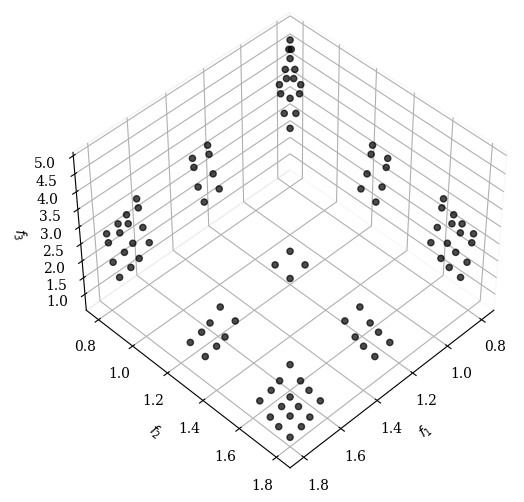
DONE
DF14¶
[14]:
from pymoo.problems.dynamic.df import DF14
import matplotlib.pyplot as plt
for t in [0.0, 0.2, 0.5, 1.0]:
plot = Scatter()
problem = DF13(time=t)
plot.add(problem.pareto_front() + 2*t, color="black", alpha=0.7)
plot.do()
plt.show()
print("DONE")
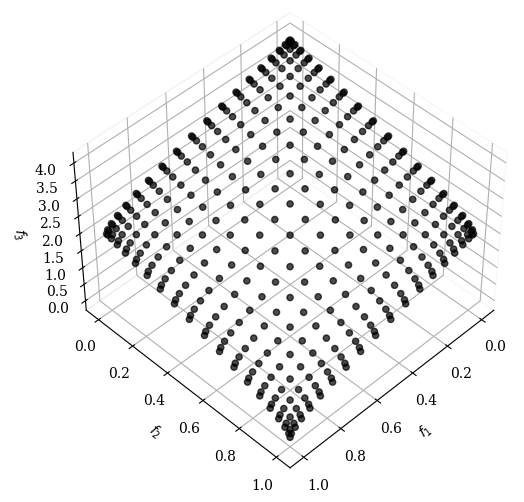
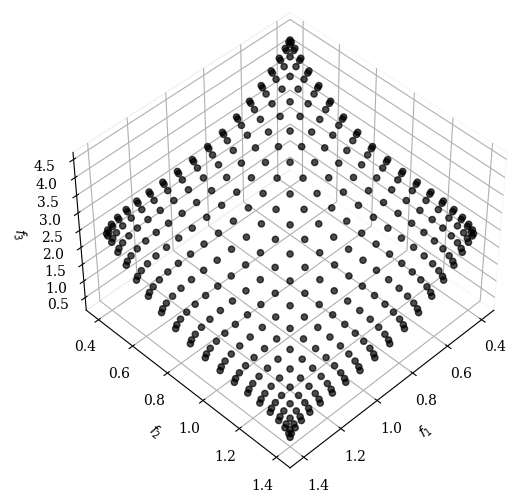
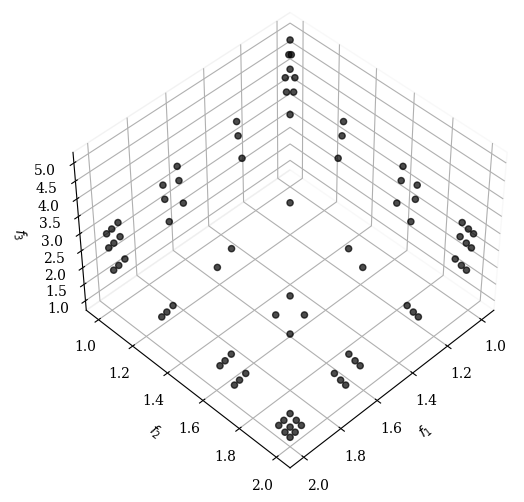
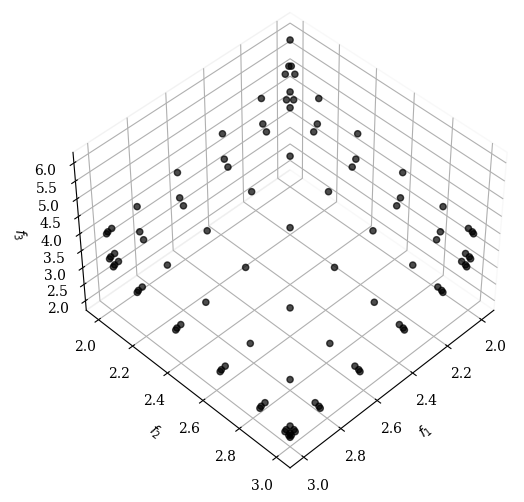
DONE