Petal Diagram¶
Also, it might be interesting to compare one solution with another. Here, a visual a single solution regarding its trade-offs.
Let us visualize some test data:
[1]:
import numpy as np
np.random.seed(1234)
F = np.random.random((1, 6))
print(F)
[[0.19151945 0.62210877 0.43772774 0.78535858 0.77997581 0.27259261]]
A simple petal plot can be created by:
[2]:
from pymoo.visualization.petal import Petal
Petal(bounds=[0, 1]).add(F).show()
[2]:
<pymoo.visualization.petal.Petal at 0x104198860>
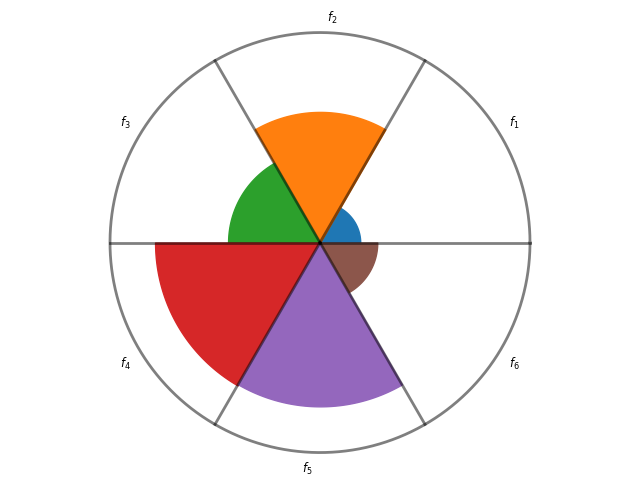
If you prefer to visualize smaller values with a larger area, set reverse=True
:
[3]:
Petal(bounds=[0, 1], reverse=True).add(F).show()
[3]:
<pymoo.visualization.petal.Petal at 0x1164acfb0>
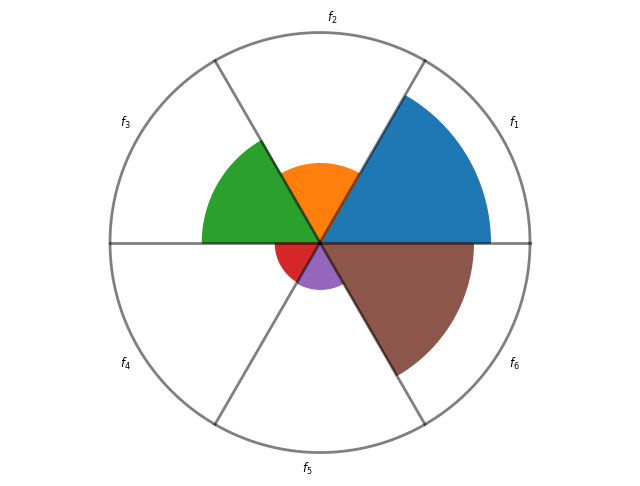
[4]:
plot = Petal(bounds=[0, 1],
cmap="tab20",
labels=["profit", "cost", "sustainability", "environment", "satisfaction", "time"],
title=("Solution A", {'pad': 20}))
plot.add(F)
plot.show()
[4]:
<pymoo.visualization.petal.Petal at 0x1163d7860>
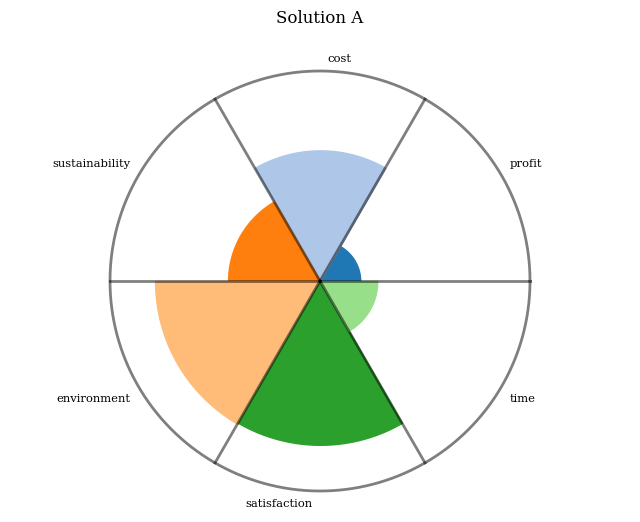
Each add will plot solutions in a row. Each entry represents a column. Easily, different solutions can be compared.
[5]:
F = np.random.random((6, 6))
plot = Petal(bounds=[0, 1], title=["Solution %s" % t for t in ["A", "B", "C", "D", "E", "F"]])
plot.add(F[:3])
plot.add(F[3:])
plot.show()
[5]:
<pymoo.visualization.petal.Petal at 0x1166490d0>
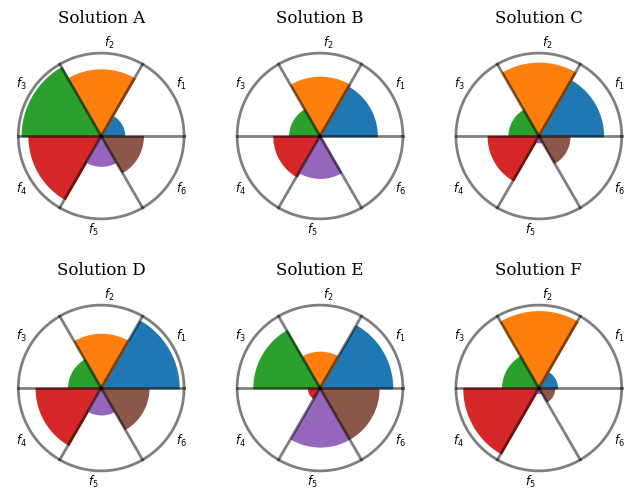
API¶
-
class
pymoo.visualization.petal.
Petal
(self, bounds=None, **kwargs) Petal Diagram
- Parameters
- boundstuple
The boundaries for each objective. Necessary to be provided for this plot!
- axis_styledict
Most of the plots consists of an axis. The style of the axis, e.g. color, alpha, …, can be changed to further modify the plot appealing.
- reversebool
Default false. Otherwise, larger area means smaller value.
- Other Parameters
- figsizetuple
The figure size. Default (figsize=(8, 6)). For some plots changing the size might have side effects for position.
- titlestr or tuple
The title of the figure. If some additional kwargs should be provided this can be achieved by providing a tuple (“name”, {“key” : val}).
- legendstr
Whether a legend should be shown or not.
- tight_layoutbool
Whether tight layout should be used.
- cmapcolormap
For some plots different kind of colors are used. The colormap can be changed to modify the color sequence for the plots.