Scatter Plot¶
The traditional scatter plot is mostly used for lower dimensional objective spaces.
Scatter 2D¶
[1]:
from pymoo.visualization.scatter import Scatter
from pymoo.problems import get_problem
F = get_problem("zdt3").pareto_front()
Scatter().add(F).show()
[1]:
<pymoo.visualization.scatter.Scatter at 0x11af31970>
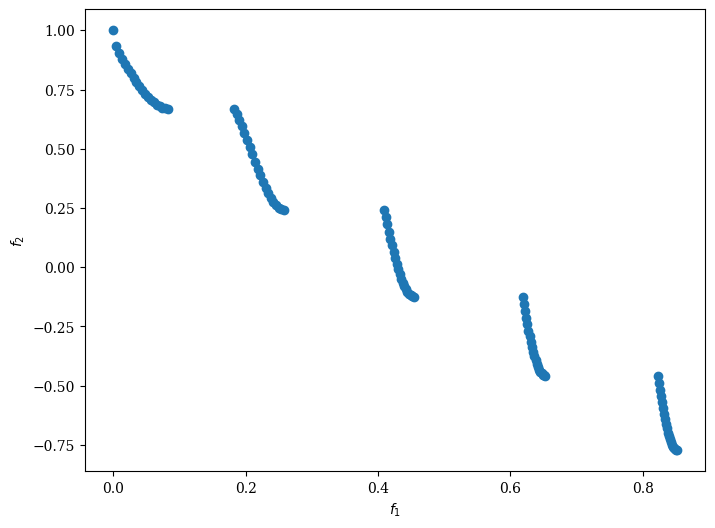
[2]:
F = get_problem("zdt3").pareto_front(use_cache=False, flatten=False)
plot = Scatter()
plot.add(F, s=30, facecolors='none', edgecolors='r')
plot.add(F, plot_type="line", color="black", linewidth=2)
plot.show()
[2]:
<pymoo.visualization.scatter.Scatter at 0x11af44cb0>
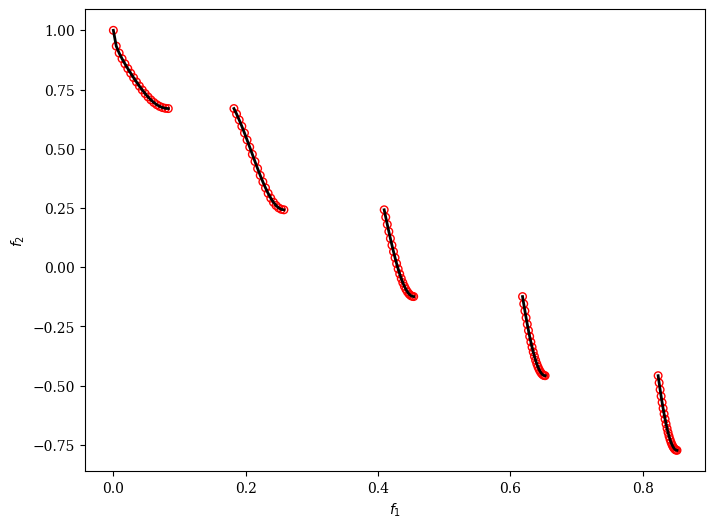
Scatter 3D¶
[3]:
from pymoo.util.ref_dirs import get_reference_directions
ref_dirs = get_reference_directions("uniform", 3, n_partitions=12)
F = get_problem("dtlz1").pareto_front(ref_dirs)
plot = Scatter()
plot.add(F)
plot.show()
[3]:
<pymoo.visualization.scatter.Scatter at 0x12ac797c0>
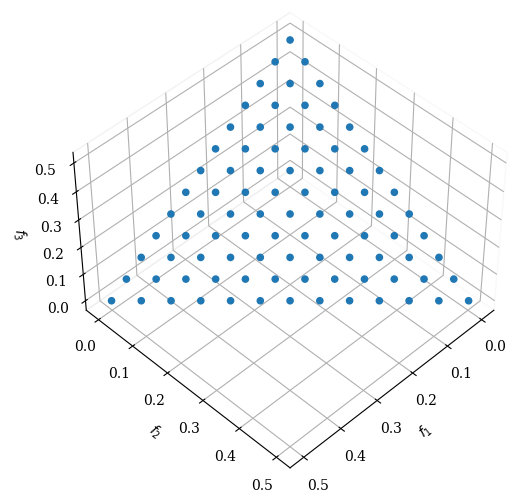
Scatter ND / Pairwise Scatter Plots¶
[4]:
import numpy as np
F = np.random.random((30, 4))
plot = Scatter(tight_layout=True)
plot.add(F, s=10)
plot.add(F[10], s=30, color="red")
plot.show()
[4]:
<pymoo.visualization.scatter.Scatter at 0x11af44ce0>
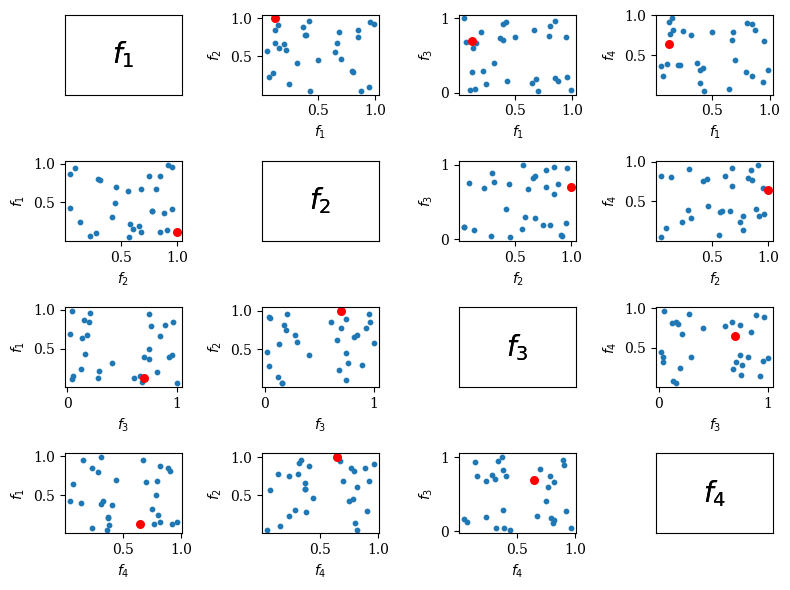
API¶
-
class
pymoo.visualization.scatter.
Scatter
(self, plot_3d=True, angle=(45, 45), **kwargs) Scatter Plot
- Parameters
- axis_styledict
Most of the plots consists of an axis. The style of the axis, e.g. color, alpha, …, can be changed to further modify the plot appealing.
- labelsstr or list
The labels to be used for each variable provided in the plot. If a string is used, then they will be enumerated. Otherwise, a list equal to the number of variables can be provided directly.
- Other Parameters
- figsizetuple
The figure size. Default (figsize=(8, 6)). For some plots changing the size might have side-effects for position.
- titlestr or tuple
The title of the figure. If some additional kwargs should be provided this can be achieved by providing a tuple (“name”, {“key” : val}).
- legendstr
Whether a legend should be shown or not.
- tight_layoutbool
Whether tight layout should be used.